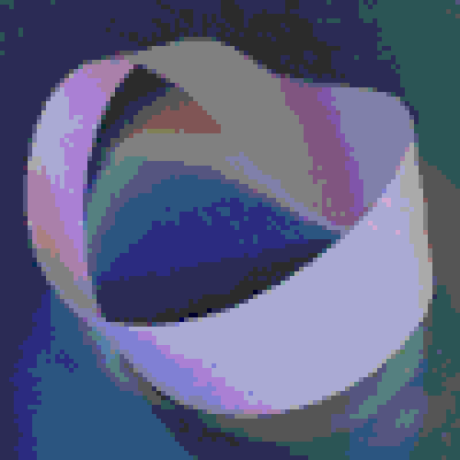
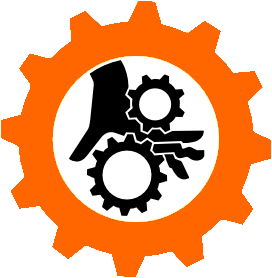
It’s also incredibly misleading, maybe it was possible to “completely” re-write the UI back in 2005—never mind that most of the value would come from, the underlying geographic data being mostly correct and mostly correctly labeled—there is no way in hell that the same would achievable in 2024. (Also the notion it would take any coder 2 * 1000 / (365 * 5/7) =
7 years to achieve a comparable result is proposterous)
I think that particular talking point also serves an exculpatory purpose: “If it was only a razor-thin victory I might understand being angry with me, but see it’s a decisive victory. He has the mandate
of heavenof the people (this is a Trumpian victory! not a Democrat failure!) ! It would be wrong not to congratulate him!”