
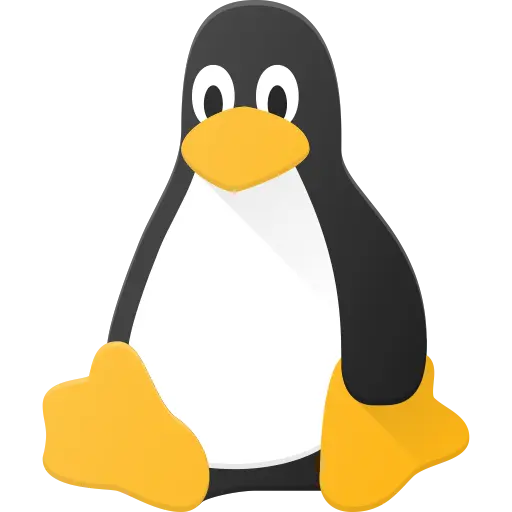
Sure, though I advice against it. The following C program can do that:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main(int argc, char **argv) {
if (argc < 2) {
fprintf(stderr, "usage: %s <command> <args>...", argv[0]);
return EXIT_FAILURE;
}
printf("Executing");
for (int i = 1; i < argc; ++i) {
printf(" %s", argv[i]);
}
puts("\nPress ^C to abort.");
sleep(5);
if (setuid(0)) {
perror("setuid");
return EXIT_FAILURE;
}
execvp(argv[1], argv + 1);
perror(argv[1]);
return EXIT_FAILURE;
}
As seen in:
$ gcc -O2 -o delay-su delay-su.c
$ sudo chown root:sudo delay-su
$ sudo chmod 4750 delay-su
$ ./delay-su id
$ id -u
1000
$ ./delay-su id -u
Executing id -u
^C to abort
0
This will allow anyone in group sudo
to execute any command as root.
You may change the group to something else to control who exactly can
run the program (you cannot change the user of the program).
If there’s some specific command you want to run, it’s better to
hard-code it or configure sudo
to allow execution of that command
without password.
Having to type
sudo
already acts as a moly-guard. Whatever OP wants to do I won’t stop them, but they are doing something strange.